We may not have the course you’re looking for. If you enquire or give us a call on 01344203999 and speak to our training experts, we may still be able to help with your training requirements.
Training Outcomes Within Your Budget!
We ensure quality, budget-alignment, and timely delivery by our expert instructors.
Exception Handling in Java is an elaborate process in which runtime errors are handled effectively. This helps maintain the application flow and results in a smooth execution. According to Oracle, Java is the number one development language for various services like microservices.
This important application requires an interruption-free flow to produce the best results. We now know that Exception Handling in Java is an effective method for handling runtime failures and preserving the application's regular flow. Further, read this blog to learn more about Java Exception Handling.
Table of Contents
1) What is an Exception in Java?
2) Why do Exceptions occur?
3) What is Exception Handling?
4) Keywords in Java Exception Handling
5) Useful methods of Exception classes
6) Java Exception Hierarchy explained
7) Types of Exceptions
8) Difference between Checked and Unchecked Exceptions
9) Conclusion
What is an Exception in Java?
In Java, an Exception is an event or condition that disrupts the normal flow of program execution. It represents an error, typically arising during runtime, that can be due to various factors such as invalid input, file not found, or network issues. Exceptions are categorised into two types: Checked Exceptions (compile-time enforced) and Unchecked Exceptions (runtime exceptions).
When an exceptional condition occurs, Java creates an Exception object containing information about the error and searches for a suitable Exception handler. Developers can use try-catch blocks to handle Exceptions, ensuring the program gracefully responds to errors. Additionally, Java's Exception hierarchy, with the base class Throwable, allows for a structured approach to handling different types of Exceptions, promoting code reliability and robust error management.
Why do Expectations occur?
Exceptions are of different types, each occurring for a different reason. The types of Exceptions will be discussed later in the blog. For now, let's run through some of the major reasons why Exceptions occur.
a) Invalid input by the user
b) Physical limitations
c) Device failure
d) Error in coding
e) Weak or no network connection
f) Opening an unavailable file
What is Exception Handling?
As explained before, Exception Handling in Java is one of the most powerful and effective methods to handle runtime errors. Handling Exceptions helps ensure the smooth and regular flow of the program. The most common runtime errors include IOException, ClassNotFoundException, SQLException, and more.
Using Exception Handling is an extremely useful method and there are various reasons for that. Some of the benefits of Exception Handling include error propagation, meaningful error reporting, and identifying error types. Additionally, handling Exceptions help in completing program execution and in the easy identification of Program Code and Error-Handling Code.
Improve your understanding of JavaScript by signing up for our JavaScript for Beginners Course now!
Keywords in Java Exception Handling
There are certain terminologies exclusively used by Java which are important to understand. Your understanding of the Exception hierarchy and Exception Handling will depend on these words. Thus, here is a list of keywords in Exception Handling:
a) try: "try" is associated with a block where the Exception codes must be placed. One of the characteristics of “try” is that the block cannot be used alone. In Java, the “try” block is always followed by a "catch" block or a "finally" block.
b) catch: "catch" refers to the block where the Exception is handled. Similar to “try”, the “catch” block also cannot be used alone. The “try” block must precede the "catch" block, or the finally block must succeed.
c) finally: The "finally" block is where the necessary code of the program is executed. A characteristic of the “finally” block is that it executes whether Exceptions are handled.
d) throw: As the name suggests, the "throw" keyword simply means to throw an Exception.
e) throws: "throws" is used to declare Exceptions. It's used alongside method signature all the time. “throws” help specify the possibility of an Exception in the method, and it doesn't throw an Exception.
f) Errors: Irreparable conditions like memory leaks, errors in stack overflow, infinite recursion, and more are represented by errors. Even the Java Virtual Machine (JVM) running out of memory falls under the abovementioned conditions.
Most of the time, the programmer cannot control the errors. Errors should not be handled by us and should not be detected, whereas Exceptions must be tried.
Useful methods of Exception classes
Java Exception and its subclasses lack specific methods, with all methods defined in the base class, Throwable. These Exception classes pinpoint different Exception scenarios for easy identification and handling. The Throwable class implements the Serializable interface for interoperability.
Key methods of the Throwable class include:
a) public String getMessage(): Returns the throwable message, set during Exception creation.
b) public String getLocalizedMessage(): Subclasses can override to provide locale-specific messages, utilising the getMessage() method.
c) public synchronized Throwable getCause(): Returns the Exception cause or null if unknown.
d) public String toString(): Returns Throwable information in String format, including class name and localised message.
e) public void printStackTrace(): Prints stack trace information to the standard error stream; writing to a file or stream with PrintStream or PrintWriter as arguments is overloaded.
Learn to make desktop applications and understand concepts of Swing. Sign up for our Java Swing Development Training now!
Java Exception Hierarchy explained
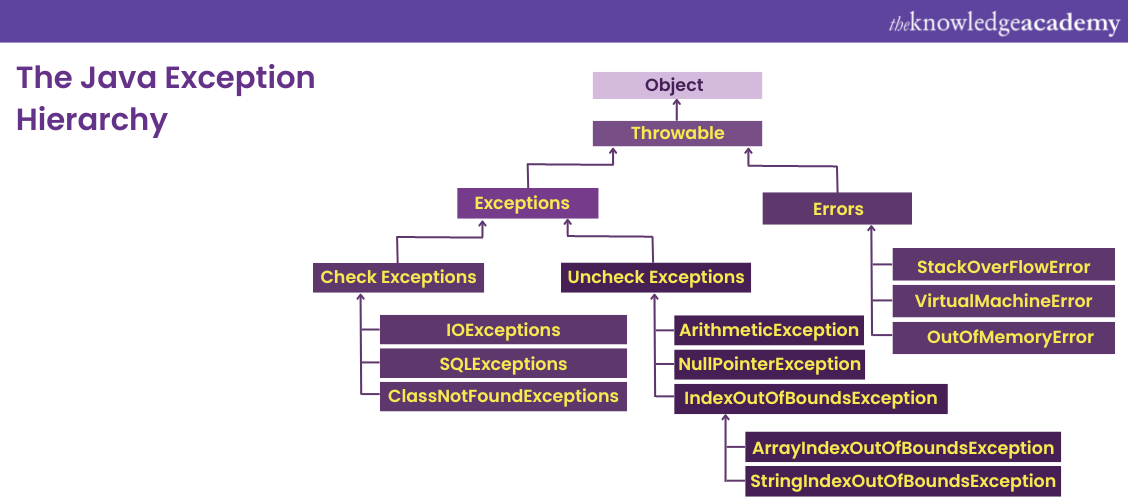
The Java Exception Hierarchy consists of classes and sub-classes that explain the position held by them. The base class of the Exception hierarchy is known as "Throwable". All the Exception types and error types act as subclasses as they fall under “Throwable” class.
The Exception class is used for the Exceptional conditions that user programs must never fail to catch. Examples of this type of Exception would be ArithmeticException or NullPointerException.
The Error class or branch is used to show errors related to the Java Run-time Environment (JRE). The Java run-time system uses errors, and the StackOverflowError can represent an example of this kind of error.
Types of Exceptions
Exceptions are mainly of two types – User-defined Exception and Built-in Exception. Built-in Exceptions are further divided into Check Exceptions and Unchecked Exceptions. It is important to understand the difference between those two.
a) Checked Exceptions: Checked Exceptions are checked during compile-time by computers. Thus, they are also known as compile-time Exceptions. Examples of checked Exceptions include IOException, SQLException, etc.
b) Unchecked Exceptions: As the name suggests, unchecked Exceptions act as the antonym for checked Exceptions. Unchecked Exceptions are not checked during compile-time.
For instance, if a program throws an unchecked Exception, regardless of whether you handle or declare it, it doesn't give out a compilation error. Examples include ArithmeticException, ArrayIndexOutOfBoundsException, etc.
Java try-catch Block
As mentioned above, the Java “try” block contains the code that could potentially throw an Exception, which must be used in a method. One of the points to remember is to not keep the code in a “try” block that will not throw an Exception. If an Exception occurs in the “try” block, the rest of the block code won't execute.
The syntax of Java try-catch is:
Try
{
//code that may throw an Exception
}catch(Exception_class_Name ref){}
The following is an example of Exception Handling using Java try-catch:
public class ExceptionHandlingExample
{
public static void main(String[] args)
{
try
{
int numerator = 10;
int denominator = 0;
int result = numerator / denominator;
System.out.println("Result: " + result);
}
catch (ArithmeticException e)
{
System.out.println("Error: Division by zero.");
}
}
}
Here, the objective is to divide the numerator by the denominator. Since the denominator is zero, it will throw an 'ArithmeticException'. The "catch” block will catch the Exception and print the error message "Error: Division by zero." to the console.
Output:
Error: Division by zero.
Java multiple catch
More than one “catch” block can succeed a “try” block, and every individual catch block must have unique Exception handlers. Thus, Java multi-catch block is used to perform different tasks upon detection of different Exceptions.
Syntax for Java multi-catch:
try
{
// code that may throw an Exception
}
catch (ExceptionType1 ExceptionVariable1)
{
// code to handle the Exception of type ExceptionType1
}
catch (ExceptionType2 ExceptionVariable2)
{
// code to handle the Exception of type ExceptionType2
}
catch (ExceptionType3 ExceptionVariable3)
{
// code to handle the Exception of type ExceptionType3
}
finally {
// code that will be executed whether an Exception was thrown or not
}
When an Exception is thrown in the “try” block, Java will check each catch block to see if it can handle it. The first “catch” block whose Exception type matches the thrown Exception will be executed, and subsequent catch blocks will be skipped.
Example of Exception Handling using Java multiple-catch
public class ExceptionHandlingExample
{
public static void main(String[] args)
{
int[] nums = {1, 2, 3, 4, 5};
try
{
System.out.println(nums[6]);
// the above line will throw an ArrayIndexOutOfBoundsException
String str = null;
System.out.println(str.length());
// the above line will throw a NullPointerException
}
catch (ArrayIndexOutOfBoundsException e)
{
System.out.println("Caught ArrayIndexOutOfBoundsException: " + e.getMessage());
}
catch (NullPointerException e)
{
System.out.println("Caught NullPointerException: " + e.getMessage());
}
catch (Exception e)
{
System.out.println("Caught Exception: " + e.getMessage()); }
finally
{
System.out.println("Finally block executed.");
}
}
}
In the above code, we create an integer array called 'nums' with five elements. In the “try” block, we attempt to print the element's value at index 6, which is outside the array's bounds and will throw an 'ArrayIndexOutOfBoundsException'. We also attempt to call the 'length' method on a null string, which will throw a 'NullPointerException'.
We use multiple catch blocks to handle these Exceptions separately. The first “catch” block catches ArrayIndexOutOfBoundsException and prints an error message. The second catch block catches NullPointerException and prints another error message. We also include a third “catch” block that catches any other Exceptions that may be thrown.
Output:
Caught ArrayIndexOutOfBoundsException: 6
Finally block executed.
Since an Exception was thrown in the first try block, the program jumps to the appropriate catch block to handle it. The finally block was also executed afterwards.
Java nested try Block
The Java nested try block is when a “try” block can be used inside another “try” block. Sometimes, a block part could cause an error, and the block itself could cause another error. In such cases, the nested try block is used as the Exception handlers need to be nested.
Syntax of Java nested try:
Try
{
// Outer try block
try {
// Inner try block
// Some code that might throw an Exception
} catch (Exception e) {
// Catch any Exception thrown in the inner try block
}
} catch (Exception e) {
// Catch any Exception thrown in the outer try block
}
Example of Exception Handling using Java nested try:
public class NestedTryExample {
public static void main(String[] args) {
int[] arr = {1, 2, 3, 4, 5};
try {
// Outer try block
for (int i = 0; i <= arr.length; i++) {
try {
// Inner try block
System.out.println(arr[i]);
} catch (ArrayIndexOutOfBoundsException e) {
// Inner catch block
System.out.println("Inner catch block: " + e);
}
}
} catch (Exception e) {
// Outer catch block
System.out.println("Outer catch block: " + e);
}
}
}
The first five lines of output correspond to the successful iteration of the for loop, printing out the values of the elements in the 'arr' array. On the 6th iteration of the for loop, we attempt to access the 6th element of the arr array, which does not exist, and this triggers an 'ArrayIndexOutOfBoundsException'.
The inner catch block catches the Exception, which prints out an error message: "Inner catch block: java.lang.ArrayIndexOutOfBoundsException: 5". Since the Exception is caught within the inner “try” block, the outer catch block is not triggered. The for loop completes, and the program ends.
Output:
1
2
3
4
5
Inner catch block: java.lang.ArrayIndexOutOfBoundsException: 5
Outer catch block: java.lang.ArrayIndexOutOfBoundsException: 5
Learn about the fundamentals of Java and programming with it. Sign up for our Java Programming Course now!
Java finally Block
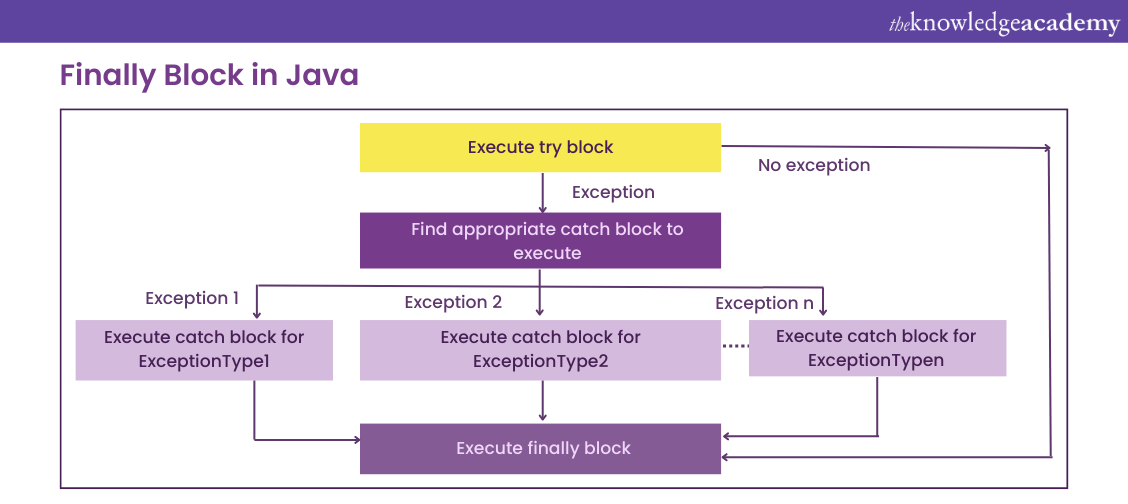
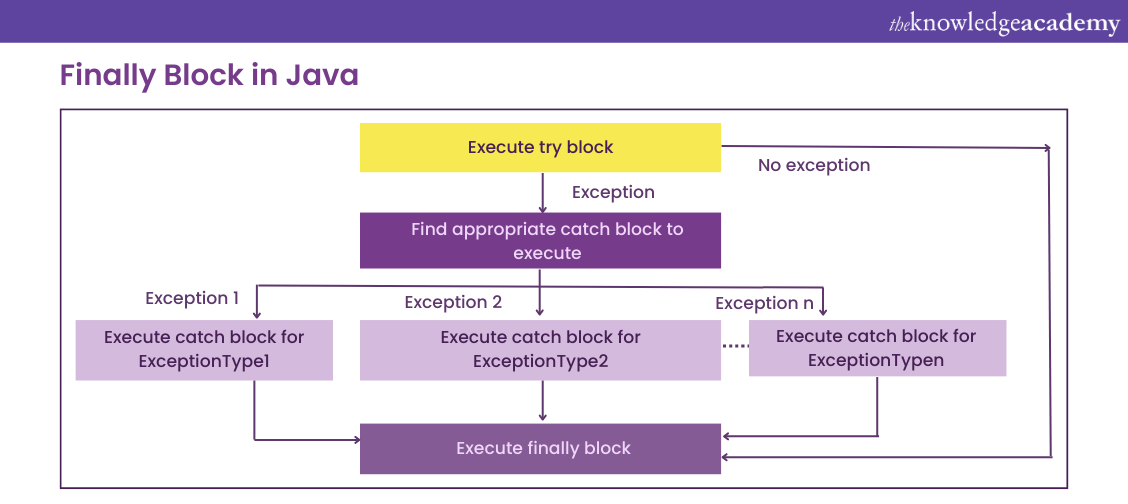
The Java “finally” block is used for the execution of important codes. One of its characteristics is that it's always executed regardless of whether an Exception is handled. This implied that it encompasses all the statements required to be printed, whether the Exception occurs.
Syntax for Java “finally” block:
try
{
// Some code that might throw an Exception
} catch (ExceptionType1 e1)
{
// ExceptionType1 handler
} catch (ExceptionType2 e2)
{
// ExceptionType2 handler
} finally
{
// Code to be executed regardless of whether an Exception was thrown or not
}
Example of Exception Handling using Java finally block:
public class Example
{
public static void main(String[] args)
{
try
{
int x = 10 / 0;
} catch (ArithmeticException e)
{
System.out.println("An error occurred: " + e.getMessage());
} finally
{
System.out.println("The finally block is always executed.");
}
}
}
In this example, we're trying to divide the integer ‘10’ by ‘0’, which is not allowed in Java and will result in an ‘ArithmeticException’. We catch the Exception and print an error message. We also have a ”finally” block that prints a message indicating that it's always executed, regardless of whether an Exception was thrown or not.
Output:
An error occurred: / by zero
The finally block is always executed.
Here, an 'ArithmeticException' is thrown and caught, and an error message is printed to the console. The 'finally' block is executed, and its message is printed to the console.
Java throw keyword
The Java “throw” keyword is used to throw an Exception explicitly, and the Exception object to be thrown is specified and has a message that describes the error. The Java “throw” keyword can throw both checked Exceptions and unchecked Exceptions.
Syntax of Java throw keyword:
throw throwable_object;
The “throw” keyword explicitly throws an Exception or error in Java. It can be used anywhere in a method, but it's typically used inside "try” blocks to handle Exceptions that the code can't handle in the "try” block.
Example of Exception Handling with Java throw keyword:
public class Example
{
public static void main(String[] args)
{
Try
{
int x = divide(10, 0);
}
catch (ArithmeticException e)
{
System.out.println("An error occurred: " + e.getMessage());
}
}
public static int divide(int a, int b)
{
if (b == 0)
{
throw new ArithmeticException("Division by zero is not allowed.");
}
return a / b;
}
}
In this example, we're trying to divide the integer '10' by '0', which is not allowed and will result in an 'ArithmeticException'. However, instead of using the / operator to perform the division, we've created a custom 'divide' method that checks whether the second argument is '0'. If it is, we throw an ArithmeticException with a custom error message.
We call the 'main' method the divide method with arguments' 10' and '0'. Since '0' is not allowed as the divisor, the divide method throws an Arithmetic Exception. We catch the Exception in the 'catch' block and print an error message to the console.
Output:
An error occurred: Division by zero is not allowed.
Difference between Checked and Unchecked Exceptions
In Java, Exceptions are classified into two main types: Checked Exceptions and Unchecked Exceptions.
Feature |
Checked Exceptions |
Unchecked Exceptions |
Compilation requirement |
Must be handled (try-catch or 'throws' clause) |
No mandatory handling, left to developer discretion |
Time of checking |
Compile-time |
Runtime |
Examples |
IOException, SQLException |
NullPointerException, ArrayIndexOutOfBoundsException |
Nature |
External factors (I/O, network) |
Logical errors in the program |
Enforcement by compiler |
Enforced by the compiler |
Not enforced by the compiler |
Handling |
Explicit handling required |
Optional handling, recommended for stability |
Flexibility |
Less flexibility due to mandatory handling |
More flexibility, but demands rigorous testing |
Typical use cases |
Network operations, file I/O |
Array access, null references |
Checked Exceptions at compile-time require the programmer to handle them explicitly. This can be done by enclosing the code that may throw the Exception in a try-catch block or by declaring the Exception in the method signature using the 'throws' clause.
Checked Exceptions typically represent external factors beyond the program's control, such as file I/O issues, network problems, or database errors. Handling them ensures a more robust and fault-tolerant program, emphasising the importance of addressing potential issues that may arise during the execution of the code.
In contrast, Unchecked Exceptions do not mandate explicit handling by the programmer. These Exceptions often result from logical errors in the program, such as attempting to access an array index that doesn't exist (ArrayIndexOutOfBoundsException) or dereferencing a null object (NullPointerException). Unchecked Exceptions are not checked by the compiler at compile-time, offering more flexibility to developers.
However, this freedom comes with the responsibility of thorough testing and code review to catch and rectify logical errors during development. While Unchecked Exceptions provide more leniency, it is crucial to adopt coding practices and testing methodologies that minimise these runtime issues, ensuring the reliability and stability of the software.
Conclusion
Exception Handling in Java is an extremely useful method to handle runtime errors. Exceptions can occur for various reasons. Thus, we hope this blog has helped you improve your understanding of handling those Exceptions.
Learn about the primary concepts of Advance Java by signing up for our Introduction to Java EE Training course now!
Frequently Asked Questions
Exception handling in Java refers to the mechanism of managing and responding to unexpected events during program execution. It involves using try-catch blocks to handle Exceptions, ensuring graceful error recovery and preventing abrupt program termination. Additionally, the 'finally' block allows for cleanup actions, enhancing code reliability.
In Java, there are three types of Exception handling:
a) try-catch: Used to enclose code that might throw Exceptions, and handles them in the catch block.
b) throws: Declares that a method may throw specific Exceptions, shifting the responsibility to the calling code.
c) finally: Encloses code that always executes, irrespective of Exceptions, facilitating cleanup operations.
The Knowledge Academy’s Knowledge Pass, a prepaid voucher, adds another layer of flexibility, allowing course bookings over a 12-month period. Join us on a journey where education knows no bounds.
The Knowledge Academy offers various Java Trainings, including Java Programming, JavaScript for Beginners and Java Swing Development Training. These courses cater to different skill levels, providing comprehensive insights into How to Become a Java Developer.
Our Programming and DevOps blogs cover a range of topics related to Java, offering valuable resources, best practices, and industry insights. Whether you are a beginner or looking to advance your Java Programming skills, The Knowledge Academy's diverse courses and informative blogs have you covered.
The Knowledge Academy takes global learning to new heights, offering over 30,000 online courses across 490+ locations in 220 countries. This expansive reach ensures accessibility and convenience for learners worldwide.
Alongside our diverse Online Course Catalogue, encompassing 17 major categories, we go the extra mile by providing a plethora of free educational Online Resources like News updates, Blogs, videos, webinars, and interview questions. Tailoring learning experiences further, professionals can maximise value with customisable Course Bundles of TKA.