Python Course Overview
Python Programming Outline
Module 1: Introduction to Python
- What is Python?
- Who Developed Python?
Module 2: Variables
- Introduction to Variables
Module 3: Data Types
- Numeric
- Boolean
- Strings
- Sequence
- Dictionary
- Set
Module 4: Operators
- Arithmetic Operators
- Assignment Operators
- Relational Operators
- Logical Operators
Module 5: Conditional Statement
- If Statement
- If-Else Statement
- Nested If Statement
- If-Elif Statement
Module 6: Looping Statement
- For Loop
- While Loop
Module 7: Functions
- Introduction to Functions
- User Defined Functions
- In-Built Functions
- Lambda Functions
Module 8: Python OOPS
- What is OOPS?
- Classes
- Objects
Module 9: Global and Local Variables
- What is a Global Variable?
- What is a Local Variable?
Module 10: Constructors
- What is a Constructor?
- Types of Constructors
Module 11: Inheritance
- What is Inheritance?
- Single Inheritance
- Multiple Inheritance
- Multi-Level Inheritance
- Hierarchical Inheritance
- Hybrid Inheritance
Module 12: Polymorphism
- What is Polymorphism?
- Method Overloading
- Method Overriding
Module 13: File Handling
- What is File Handling?
- Open
- Write
- Append
Module 14: Exception Handling
- What is Exception Handling?
- Difference Between Syntax Error and Exceptions
- Components of Exception Handling
- Try Block
- Except Block
- Else Block
- Finally Block
- Raise Keyword
Module 15: Modules
- What is Module?
- Module Creation
- Module Importing
Module 16: Packages
- What is a Package?
- Creation of Package
- Importing Modules from Packages
- Importing Specific Function from Module
Module 17: Web Scrapping
- What is Web Scrapping?
- Requests Module
- BeautifulSoup Library
Module 18: NumPy
- What is NumPy?
- Installation of NumPy
- Operation in NumPy
Module 19: Python with MySQL
- Environment Setup
- Creating Database Connection
- Creating Databases and Tables in Python
- Operations on Tables in Python
Module 20: Python GUI Tkinter
- Python GUI Application with Tkinter
- Widgets
- Getting User Input
- Assigning Widgets to Frames
- Controlling Layout with Geometry Managers
- pack()
- place()
- grid()
Module 21: Python Newspaper
- Newspaper Module
- Installation
- Implementation
Module 22: Ping-Pong Game
- What is a Ping-Pong Game?
- Working with Turtle Module
- Creating Right, Left Paddles, and a Ball
Who should attend this Python Course?
This Python Training Course will provide a comprehensive understanding to individuals who want to learn Coding using the Python programming language. This course can be beneficial to a wide range of professionals, including:
- Software Developers
- Data Analysts
- Web Developers
- Data Scientists
- Systems Analysts
- IT Professionals
- Software Engineers
Prerequisites of the Python Course
There are no formal prerequisites to attend the Python Course. However, a basic understanding of different programming languages would be beneficial for delegates.
Python Course Overview
Python Programming is a fundamental skill offering immense relevance in today's technology-driven landscape. This versatile language is known for its readability and ease of use, making it a popular choice for beginners and experienced programmers alike. With its wide range of applications in web development, data analysis, and more, proficiency in Python is a valuable asset for anyone interested in Programming.
Proficiency in Python Programming is vital for professionals in various fields, including Software Development, Data Science, and Artificial Intelligence. It empowers professionals to build efficient, scalable, and innovative solutions. As the demand for Programming Courses continues to rise, mastering Python becomes essential for those aiming to excel in their careers and stay competitive in the rapidly evolving tech industry.
This intensive 2-day Python Course equips delegates with essential skills and knowledge. Through hands-on exercises, real-world examples, and expert guidance, delegates will gain a solid foundation in Python Programming. This training enhances problem-solving abilities and fosters creativity, ensuring delegates are well-prepared to tackle real-world challenges.
Course Objectives:
- To understand Python syntax and its fundamental concepts
- To learn to develop Python applications for various domains
- To gain proficiency in data manipulation and analysis using Python
- To explore web development and automation with Python
- To master object-oriented programming techniques in Python
- To enhance problem-solving skills and algorithmic thinking
- To create and deploy Python applications in practical scenarios
After completing this course, delegates will have the knowledge and confidence to embark on a journey of creating innovative solutions. They will be ready to apply their skills in diverse professional settings and explore these advanced courses, leveraging Python as a solid foundation for their careers.
What’s included in this Python Course?
- World-Class Training Sessions from Experienced Instructors
- Python Programming Certificate
- Digital Delegate Pack
Why choose us
Ways to take this course
Experience live, interactive learning from home with The Knowledge Academy's Online Instructor-led Python Course. Engage directly with expert instructors, mirroring the classroom schedule for a comprehensive learning journey. Enjoy the convenience of virtual learning without compromising on the quality of interaction.
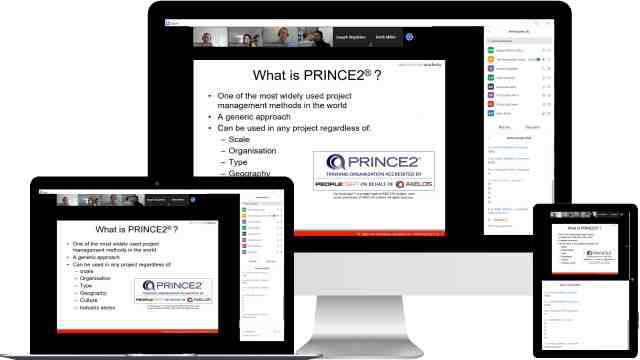
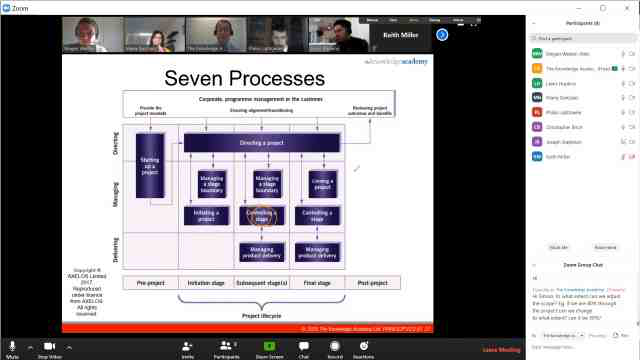
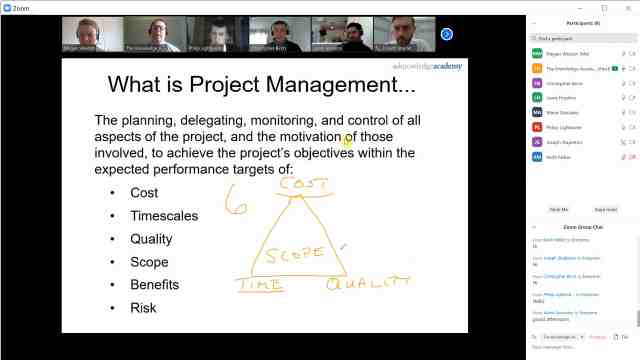
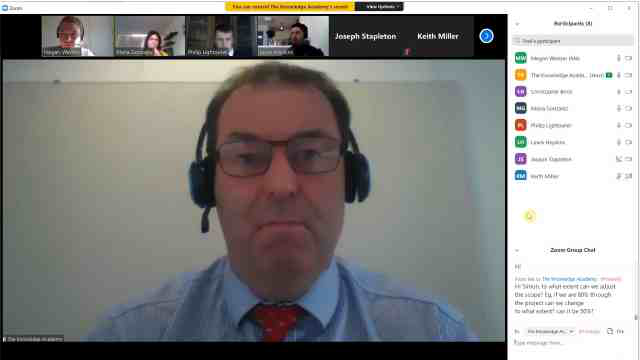
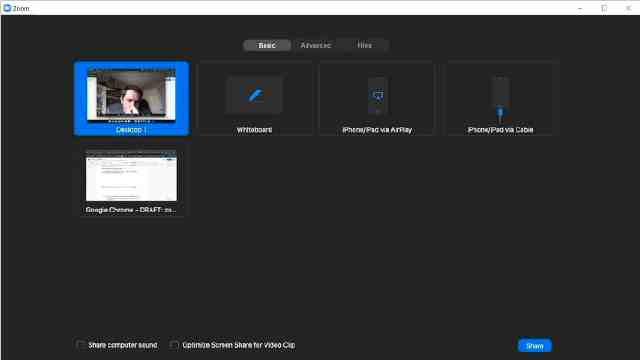
Unlock your potential with The Knowledge Academy's Python Course, accessible anytime, anywhere on any device. Enjoy 90 days of online course access, extendable upon request, and benefit from the support of our expert trainers. Elevate your skills at your own pace with our Online Self-paced sessions.
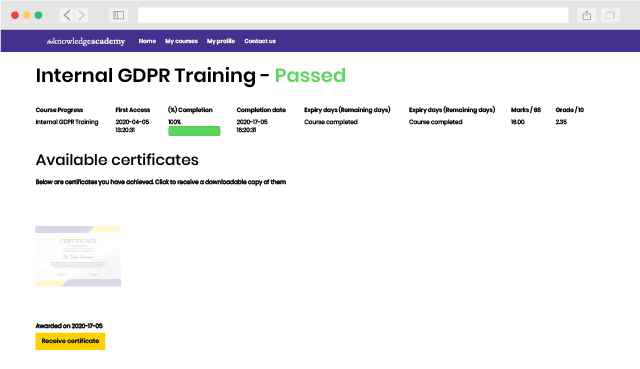
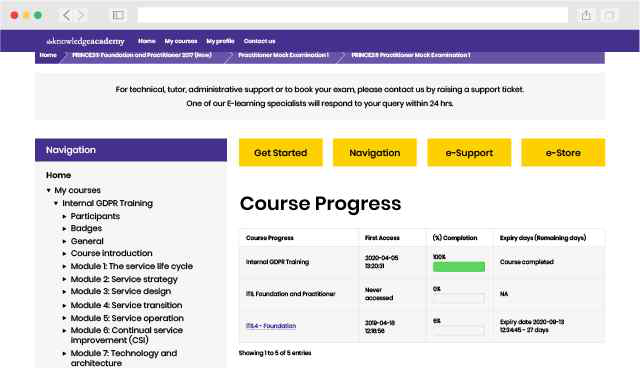
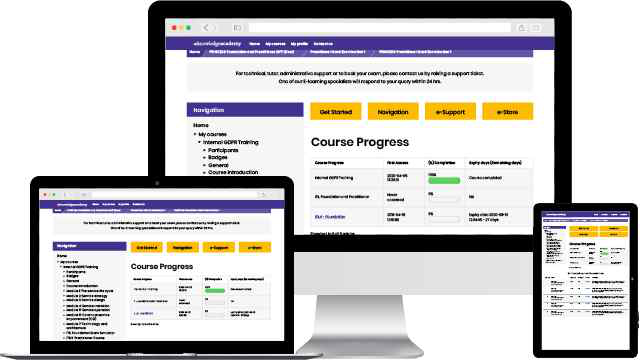
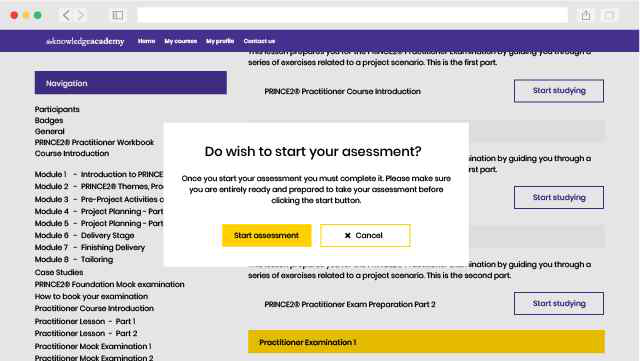
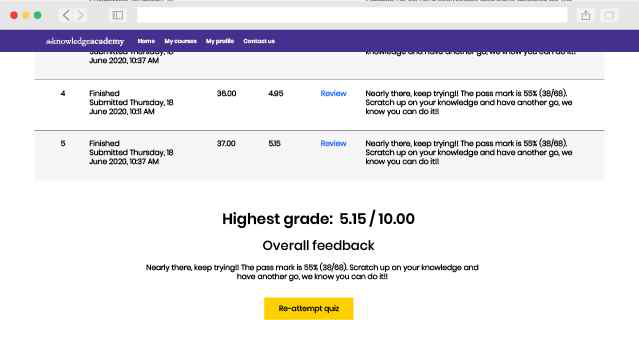
Experience the most sought-after learning style with The Knowledge Academy's Python Course. Available in 490+ locations across 190+ countries, our hand-picked Classroom venues offer an invaluable human touch. Immerse yourself in a comprehensive, interactive experience with our expert-led Python Course sessions.

Highly experienced trainers
Boost your skills with our expert trainers, boasting 10+ years of real-world experience, ensuring an engaging and informative training experience

State of the art training venues
We only use the highest standard of learning facilities to make sure your experience is as comfortable and distraction-free as possible

Small class sizes
Our Classroom courses with limited class sizes foster discussions and provide a personalised, interactive learning environment

Great value for money
Achieve certification without breaking the bank. Find a lower price elsewhere? We'll match it to guarantee you the best value
Streamline large-scale training requirements with The Knowledge Academy’s In-house/Onsite Python Course at your business premises. Experience expert-led classroom learning from the comfort of your workplace and engage professional development.

Tailored learning experience
Leverage benefits offered from a certification that fits your unique business or project needs

Maximise your training budget
Cut unnecessary costs and focus your entire budget on what really matters, the training.

Team building opportunity
Our Python Course offers a unique chance for your team to bond and engage in discussions, enriching the learning experience beyond traditional classroom settings
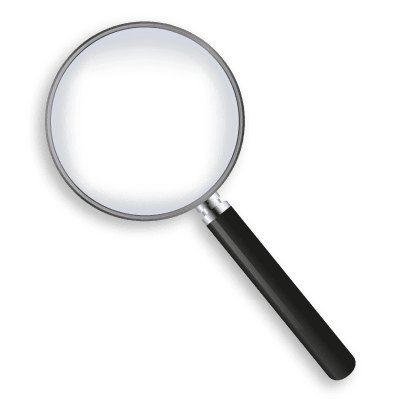
Monitor employees progress
The course know-how will help you track and evaluate your employees' progression and performance with relative ease
What our customers are saying
the trainer is very helpful and patient with material
Chao William Thao
very likely, Priyanka did a great job explaining everything in a simple way and the course was really interavtive.
Michail Tegos
Trainer possesses excellent knowledge, and I thoroughly enjoyed the training with him.
Muhammad Adil
Python Course FAQs
Why choose us
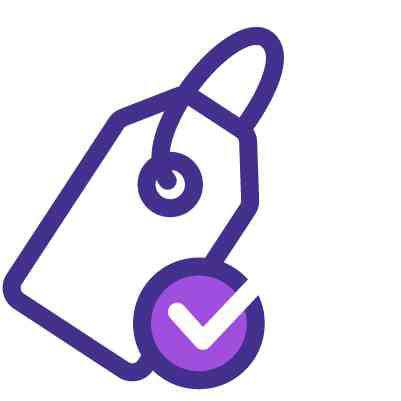
Best price in the industry
You won't find better value in the marketplace. If you do find a lower price, we will beat it.
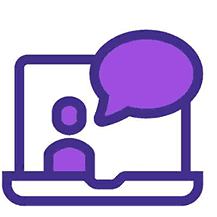
Many delivery methods
Flexible delivery methods are available depending on your learning style.
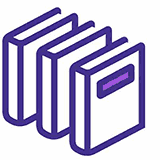
High quality resources
Resources are included for a comprehensive learning experience.
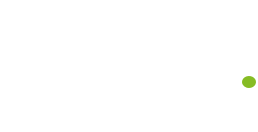
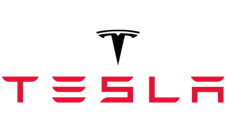
"Really good course and well organised. Trainer was great with a sense of humour - his experience allowed a free flowing course, structured to help you gain as much information & relevant experience whilst helping prepare you for the exam"
Joshua Davies, Thames Water
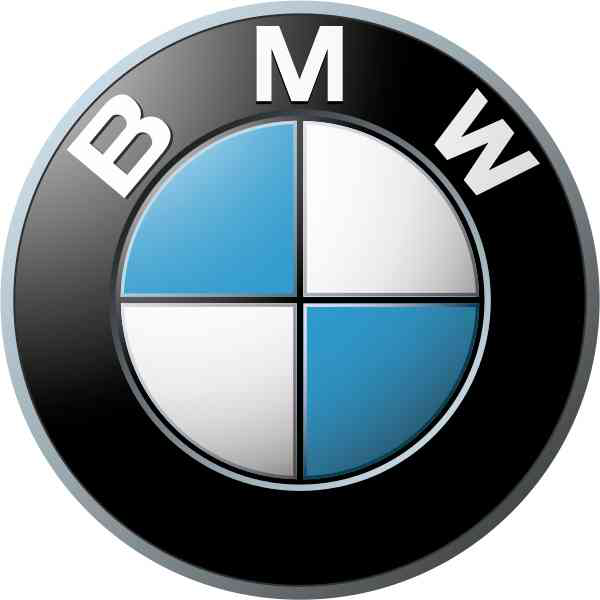
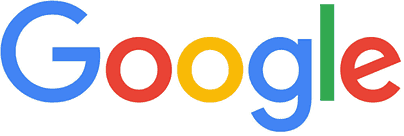
Looking for more information on Programming Training?
Programming Bundle
Save upto 40%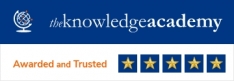
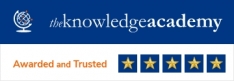
Total without package: $4590
Package price: $2795 (Save $1795)